Using jQuery stringify plugin is an easy way to display a json string (curly bracket javascript object text) to solve programing problems. You might have received invalid json string error or you are having a hard time displaying info from a json string you were receiving as a response into your code.
Json stringiyfy extension is
found here ( https://gist.github.com/chicagoworks/754454 ) and JQuery library can be
downloaded here (http://code.jquery.com/jquery-2.0.3.min.js)
Just need to copy paste Stringify plugin either in a external text file with .js extension or directly inside script tag. In this example I copy paste Strigify plugin in a script tag right below jQuery library script.
I've created a sample of html file and pasted here to show where all the script tags go.
<!DOCTYPE html>
<html>
<head>
<title></title>
<script src="jquery-2.0.3.min.js"></script>
<script type="text/javascript">
//this is jquery stringify plugin for debugging purposes..example usage: jQuery.stringify(Place-The-Object-Variable-Here);
//begin jQuery stringify plugin
jQuery.extend({
stringify : function stringify(obj) {
var t = typeof (obj);
if (t != "object" || obj === null) {
// simple data type
if (t == "string") obj = '"' + obj + '"';
return String(obj);
} else {
// recurse array or object
var n, v, json = [], arr = (obj && obj.constructor == Array);
for (n in obj) {
v = obj[n];
t = typeof(v);
if (obj.hasOwnProperty(n)) {
if (t == "string") v = '"' + v + '"'; else if (t == "object" && v !== null) v = jQuery.stringify(v);
json.push((arr ? "" : '"' + n + '":') + String(v));
}
}
return (arr ? "[" : "{") + String(json) + (arr ? "]" : "}");
}
}
});
//end stringify plugin
//json object to test with
var ItemsToMakeSandwich = {
Bread: {slices: 2 },
"Peanut Butter": {jar: 1},
Jam: {jar: 1}
};
</script>
</head>
<body>
<h4>With JQuery Stringify</h4>
<p id="message"> </p>
<h4>Without JQuery Stringify</h4>
<p id="messagetwo"> </p>
<script type="text/javascript">
//this selects the p tag with id message and replaces text inside
$("#message").text(""+ jQuery.stringify(ItemsToMakeSandwich) +"");
//this selects the p tag with id messagetwo and replaces text inside
$("#messagetwo").text(""+ ItemsToMakeSandwich +"");
</script>
</body>
</html>
This code converts Javascript JSON to string:
jQuery.stringify(ItemsToMakeSandwich);
You need to have stringify plugin to use "jQuery.stringify()".
Here is browser screenshot results of above html and javascript showing an example of JSON String:
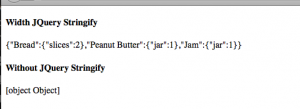
We can see how useful jQuery Stringify plugin is when debugging javascript code being used to program with a cloud API such as kinvey. For example you can do an alert against a json response being sent by Kinvey or any other cloud service. This helps in seeing how your code should be traversing received json object.
alert(""+ jQuery.stringify(response) +"") +"");
You can also see json objects in Firefox firebug or Chrome developer tools.